What is Pagination in Web Development?
ghifari
July 29, 2024
6 min read
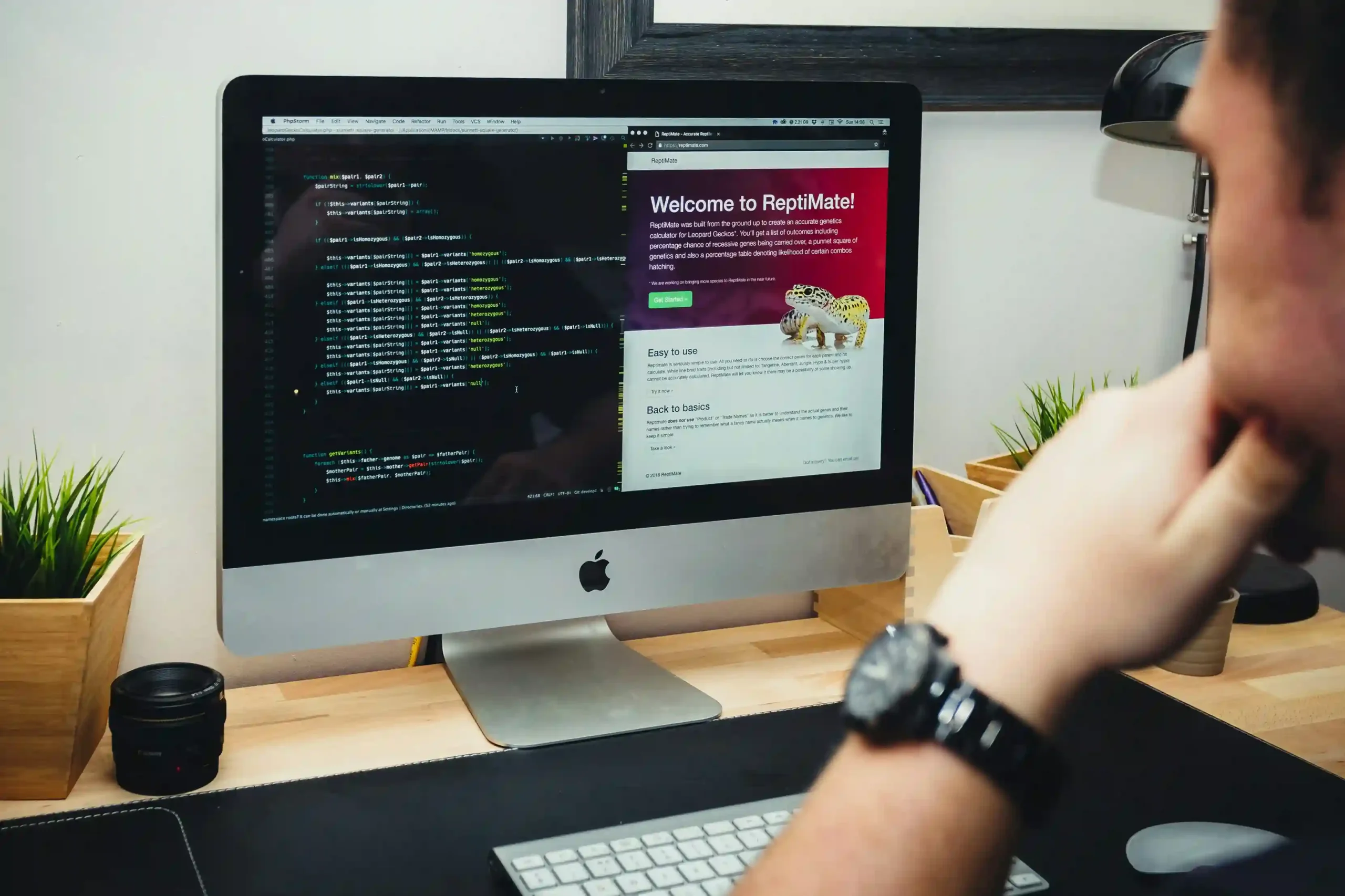
Pagination is a crucial concept in web development that significantly enhances the user experience and improves website functionality. If you’ve ever browsed an online store or read through a blog with multiple pages of posts, you’ve encountered pagination. In essence, pagination divides content across multiple pages, making it easier for users to navigate through large amounts of data without being overwhelmed by a single, lengthy page.
Why is Pagination Important?
Pagination is essential for several reasons:
- Improves User Experience: By breaking down content into manageable chunks, pagination helps users find what they’re looking for more efficiently. It also reduces the cognitive load, making the browsing experience more pleasant.
- Enhances Performance: Loading a vast amount of content on a single page can slow down a website. Pagination allows for faster load times by only displaying a subset of data at any given time.
- SEO Benefits: Search engines prefer well-structured and organized content. Properly implemented pagination can help with indexing and can distribute page authority across multiple pages.
How Does Pagination Work?
Pagination involves several elements:
- Page Numbers: Typically found at the bottom of a webpage, page numbers allow users to navigate through different sections of content. Clicking on a number takes the user to the corresponding page.
- Next and Previous Buttons: These buttons enable users to move sequentially through the content, one page at a time.
- Load More Button: Instead of traditional pagination, some websites use a “load more” button that loads additional content on the same page when clicked.
Implementing Pagination in Web Development
Pagination can be implemented in various ways, depending on the platform and programming language. Here’s a breakdown of common methods:
Using HTML and CSS
The simplest form of pagination can be achieved using HTML and CSS. Here’s an example:
<div class="pagination">
<a href="#">« Previous</a>
<a href="#">1</a>
<a href="#">2</a>
<a href="#">3</a>
<a href="#">Next »</a>
</div>
.pagination {
display: flex;
justify-content: center;
margin: 20px 0;
}
.pagination a {
margin: 0 5px;
padding: 8px 16px;
text-decoration: none;
color: #333;
background-color: #f1f1f1;
border: 1px solid #ddd;
}
.pagination a:hover {
background-color: #ddd;
}
This code creates a simple pagination bar with clickable page numbers and next/previous buttons.
Using JavaScript
For more dynamic pagination, JavaScript is often used. This approach can fetch and display new content without reloading the entire page.
document.addEventListener('DOMContentLoaded', () => {
const pagination = document.querySelector('.pagination');
pagination.addEventListener('click', (e) => {
if (e.target.tagName === 'A') {
e.preventDefault();
const page = e.target.textContent.trim();
loadPage(page);
}
});
function loadPage(page) {
// Fetch content for the given page
fetch(`/content?page=${page}`)
.then(response => response.text())
.then(data => {
document.querySelector('.content').innerHTML = data;
});
}
});
This script listens for clicks on the pagination links and fetches new content based on the page number.
Using Server-Side Languages
Server-side pagination is handled on the backend using languages like PHP, Python, or Node.js. Here’s an example with PHP:
<?php
$host = 'localhost';
$db = 'your_database';
$user = 'your_username';
$pass = 'your_password';
$dsn = "mysql:host=$host;dbname=$db";
$options = [
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION,
PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC,
];
$pdo = new PDO($dsn, $user, $pass, $options);
$limit = 10; // Number of entries to show in a page.
if (isset($_GET["page"])) {
$page = $_GET["page"];
} else {
$page=1;
};
$start_from = ($page-1) * $limit;
$sql = "SELECT * FROM your_table LIMIT $start_from, $limit";
$rs_result = $pdo->query($sql);
?>
<!DOCTYPE html>
<html>
<head>
<title>Pagination</title>
</head>
<body>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
</tr>
</thead>
<tbody>
<?php
while ($row = $rs_result->fetch()) {
echo "<tr>
<td>" . $row["id"] . "</td>
<td>" . $row["name"] . "</td>
</tr>";
};
?>
</tbody>
</table>
<div class="pagination">
<?php
$sql = "SELECT COUNT(id) FROM your_table";
$rs_result = $pdo->query($sql);
$row = $rs_result->fetch();
$total_records = $row[0];
$total_pages = ceil($total_records / $limit);
for ($i=1; $i<=$total_pages; $i++) {
echo "<a href='index.php?page=".$i."'>".$i."</a> ";
};
?>
</div>
</body>
</html>
This PHP script fetches a specific number of records from a database and displays them with pagination links.
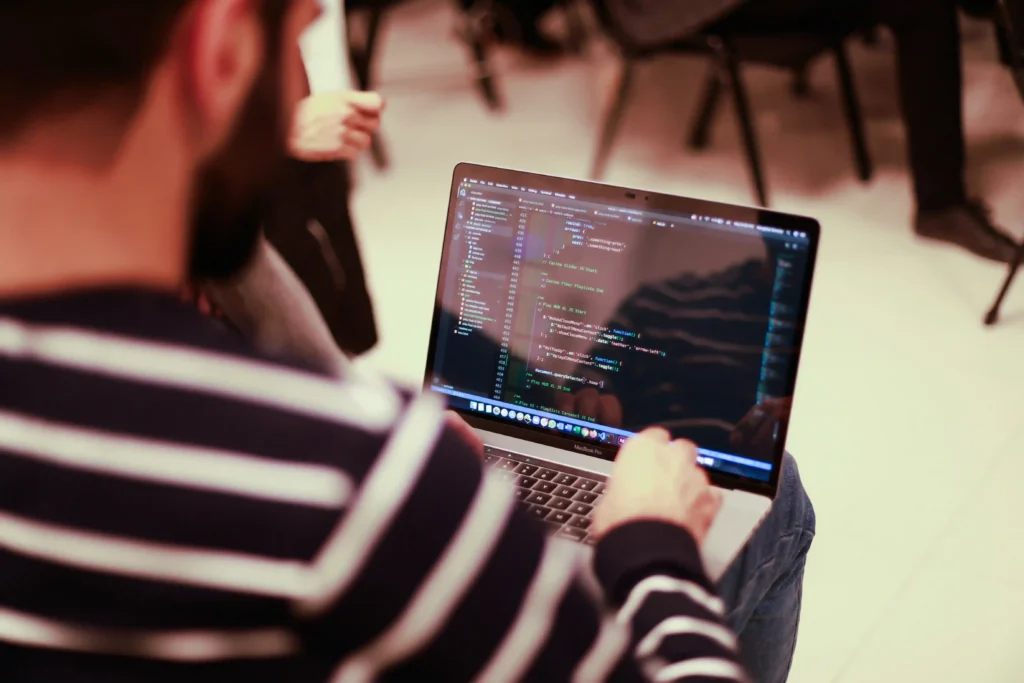
Best Practices for Pagination
Implementing pagination effectively requires adherence to several best practices:
- Consistent Design: Ensure that pagination controls are easy to find and use. They should be consistently styled and placed in a predictable location on the page.
- Clear Labels: Use clear and descriptive labels for pagination controls. Instead of just “Next” and “Previous,” consider adding more context like “Next Page” or “Page 2 of 10.”
- Accessibility: Make sure that pagination controls are accessible to all users, including those using screen readers. Use appropriate ARIA roles and labels.
- SEO-Friendly URLs: Ensure that pagination links use clean, descriptive URLs. Avoid using parameters that are hard to read or understand.
- Performance Optimization: Optimize the backend to handle pagination efficiently, especially for large datasets. Use indexed queries and caching where appropriate.
Common Challenges with Pagination
While pagination is beneficial, it can present some challenges:
- Infinite Scroll vs. Pagination: Some websites opt for infinite scroll instead of traditional pagination. While infinite scroll can enhance the user experience for certain types of content (e.g., social media feeds), it can be detrimental for others, making it hard to reach footer content or specific pages.
- SEO Considerations: Improperly implemented pagination can lead to duplicate content issues and poor indexing by search engines. Use rel=”next” and rel=”prev” tags to help search engines understand the relationship between paginated pages.
- Load Performance: If not optimized, paginated queries can slow down the website, especially for large datasets. Always ensure your database queries are efficient and that the server can handle the load.
Conclusion
Pagination is a fundamental aspect of web development that plays a critical role in enhancing user experience, improving website performance, and aiding in SEO efforts. By understanding and implementing effective pagination strategies, developers can create more user-friendly and efficient websites. Whether using simple HTML and CSS, dynamic JavaScript, or server-side scripting, the key is to keep the user experience at the forefront while ensuring optimal performance and search engine compatibility.
Need Help with Web Development?
At Available Dev, we specialize in creating high-performance, user-friendly websites. Whether you need assistance with pagination, SEO, or any other aspect of web development, our expert team is here to help. Contact us today to see how we can elevate your online presence and provide a seamless browsing experience for your users.
Visit Available Dev to learn more about our web development services!
Related Article
How to Choose the Best Web Development Framework for Your Project
In the fast-paced world of web design, choosing the right web... In the fast-paced world of web design, choosing the right web development framework can make or break your project. With countless options...
10 Essential Skills Every Web Developer Should Learn
Photo by Joshua Aragon on Unsplash In today’s tech-driven world,... Photo by Joshua Aragon on Unsplash In today’s tech-driven world, web development is one of the most in-demand careers. Whether...
Top Web Development Trends in 2024: What’s New in Tech?
Photo by Andrew Neel on Unsplash The web development landscape... Photo by Andrew Neel on Unsplash The web development landscape is constantly evolving, and 2024 is shaping up to be...