What is Prisma Used for in Web Development?
ghifari
August 9, 2024
8 min read
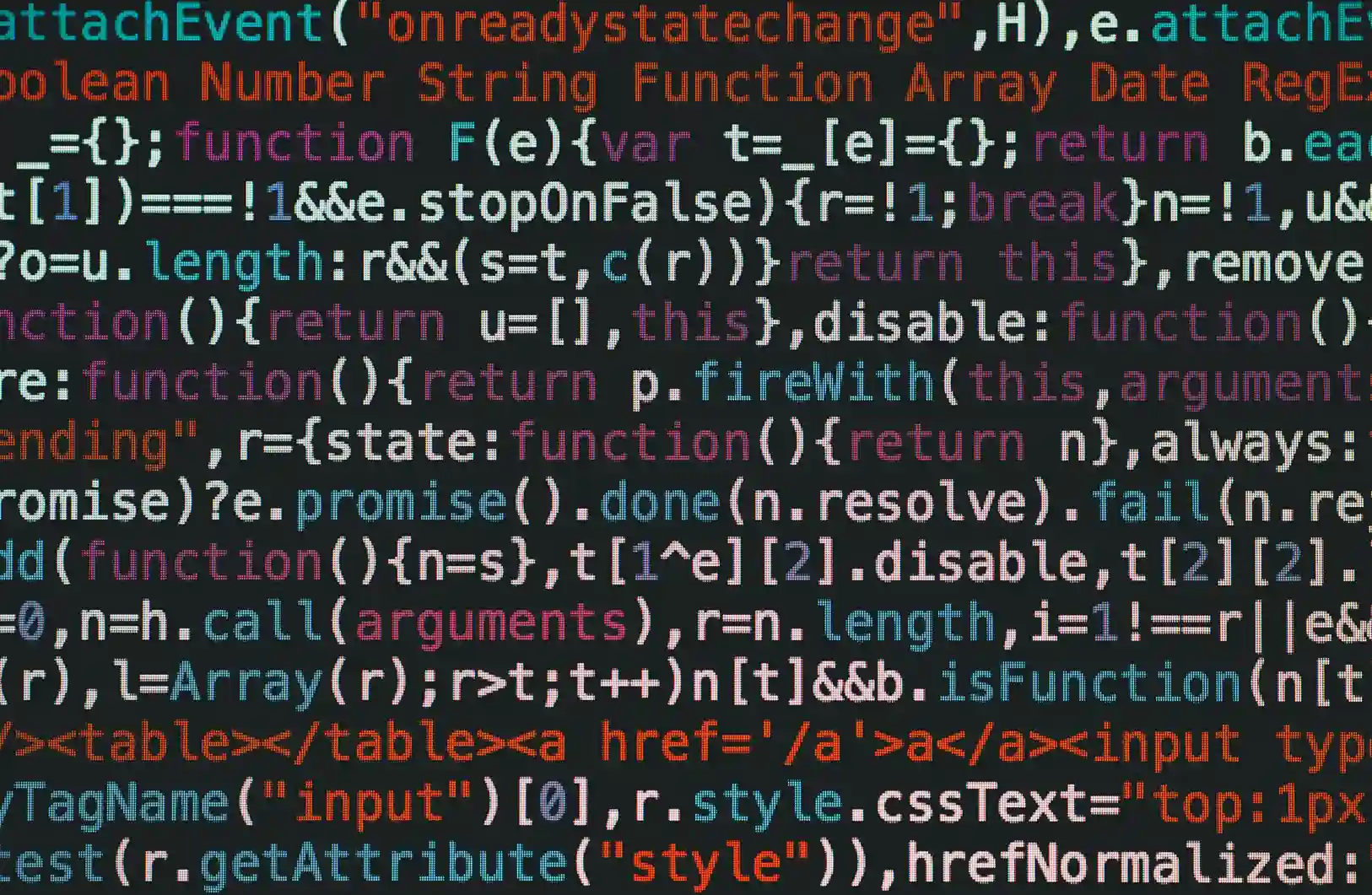
In the ever-evolving world of web development, developers constantly search for tools that can make their work easier, faster, and more efficient. One such tool that has gained significant attention is Prisma. If you’ve been wondering what Prisma is and how it can be used in web development, you’ve come to the right place. This article will explore Prisma, its benefits, and how it’s used to improve web development workflows.
Understanding Prisma: What Is It?
Prisma is an open-source next-generation Node.js and TypeScript ORM (Object-Relational Mapping) that simplifies database management for developers. Traditionally, interacting with databases requires writing raw SQL queries, which can be cumbersome, error-prone, and difficult to manage, especially as your application grows. Prisma abstracts much of this complexity, providing a more intuitive and type-safe way to work with databases.
Prisma consists of three main components:
- Prisma Client: A type-safe query builder that allows you to interact with your database in a more structured and efficient way.
- Prisma Migrate: A migration tool that helps manage and version database schema changes.
- Prisma Studio: A visual database interface that makes it easy to interact with your database, browse data, and debug issues.
These components work together to streamline database operations, making it easier to build and maintain web applications.
The Benefits of Using Prisma
1. Type Safety and Autocompletion
One of Prisma’s standout features is its type safety. Prisma Client is generated based on your database schema, meaning it knows the exact structure of your database. This allows it to provide powerful autocompletion and type-checking capabilities in your code editor, significantly reducing the likelihood of runtime errors due to incorrect database queries.
For example, if you have a table named Users
with columns id
, name
, and email
, Prisma Client will automatically generate TypeScript types for this table. When you query the Users
table in your application, you’ll get type-safe queries that ensure you’re accessing the correct columns and data types.
2. Ease of Use
Prisma abstracts much of the complexity associated with traditional ORMs and raw SQL queries. It provides a clean and intuitive API that is easy to learn and use, even for developers who may not be database experts. This ease of use extends to managing relationships between tables, handling complex queries, and performing CRUD (Create, Read, Update, Delete) operations.
For example, to fetch all users from the Users
table, you can simply write:
const users = await prisma.user.findMany();
This simplicity allows developers to focus more on building features rather than worrying about database intricacies.
3. Migration Management
Database schema changes are inevitable as your application evolves. However, managing these changes can be challenging, especially when working in a team or deploying to multiple environments. Prisma Migrate provides a robust solution for managing database migrations.
With Prisma Migrate, you can create migration files that describe changes to your database schema, such as adding or removing columns, renaming tables, or altering data types. These migration files can be versioned and applied across different environments, ensuring consistency and reducing the risk of errors during deployment.
4. Performance Optimization
Prisma is designed with performance in mind. It generates optimized SQL queries that are tailored to your database schema and the specific operations you’re performing. This means you can achieve faster query execution times and reduce the load on your database, resulting in a more responsive application.
Additionally, Prisma supports connection pooling and lazy loading, further improving the performance of your application by efficiently managing database connections and fetching only the data you need.
5. Multi-Databases and Database Agnosticism
Prisma supports multiple databases, including PostgreSQL, MySQL, SQLite, SQL Server, and MongoDB (currently in preview). This flexibility allows you to choose the database that best suits your needs while maintaining a consistent development experience across different projects.
Prisma’s database-agnostic approach means that you can switch between supported databases with minimal changes to your application code. This is particularly useful if your project requirements change or if you need to support multiple databases in a single application.
6. Improved Collaboration
Prisma’s clear and consistent API makes it easier for teams to collaborate on web development projects. The auto-generated types and autocompletion features help ensure that everyone on the team is on the same page when it comes to interacting with the database. This reduces the likelihood of miscommunication and errors, leading to smoother and more efficient development processes.
Additionally, Prisma’s migration tools make it easier to coordinate schema changes across a team, ensuring that everyone is working with the same version of the database schema.
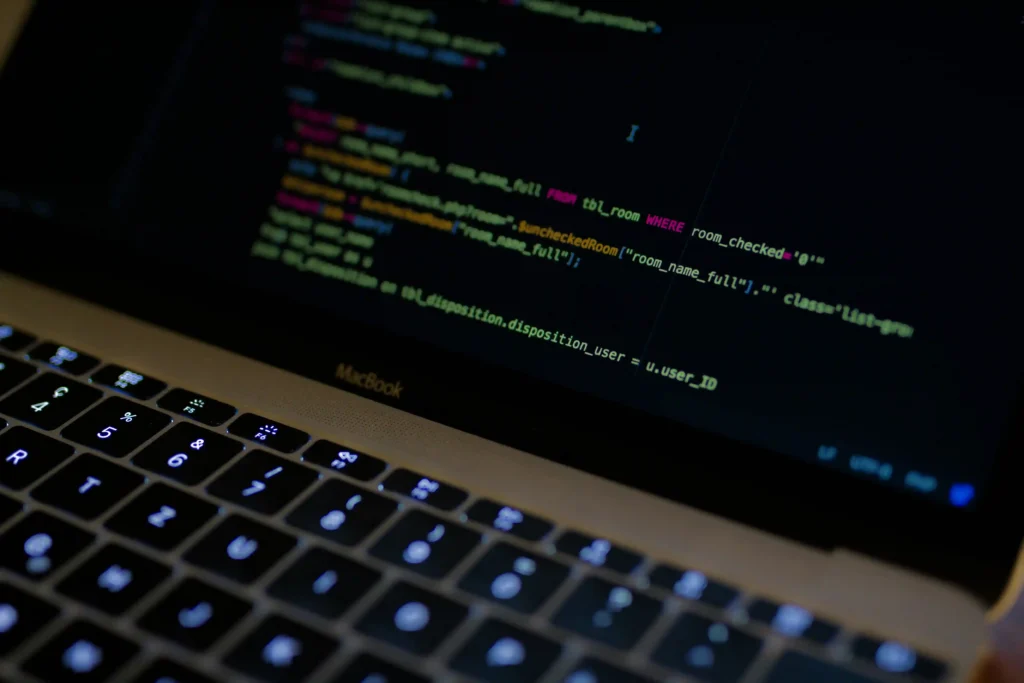
How Prisma Is Used in Web Development
1. Building APIs
One of the most common use cases for Prisma in web development is building APIs. Whether you’re developing a RESTful API or a GraphQL API, Prisma simplifies the process of interacting with your database. It allows you to write concise and readable code for fetching, creating, updating, and deleting data, all while ensuring type safety.
For example, when building a GraphQL API with Prisma, you can use the Prisma Client to resolve queries and mutations efficiently. The type-safe nature of Prisma ensures that your GraphQL schema and resolvers align perfectly with your database schema, reducing the chances of runtime errors.
2. Developing Server-Side Applications
Prisma is also widely used in developing server-side applications, particularly those built with frameworks like Express.js, Next.js, and NestJS. By integrating Prisma into your server-side code, you can handle database interactions more effectively, whether you’re working on a small personal project or a large-scale enterprise application.
Prisma’s ease of use and powerful querying capabilities make it an excellent choice for handling the backend logic of your web application. You can efficiently manage user authentication, handle form submissions, process transactions, and more, all with minimal boilerplate code.
3. Prototyping and Rapid Development
When working on a new project or feature, rapid prototyping is often essential to validate ideas quickly. Prisma’s intuitive API and migration tools make it easy to set up and iterate on your database schema, allowing you to build and test features rapidly.
Whether you’re developing a new module or adding functionality to an existing application, Prisma enables you to move quickly while maintaining code quality and database integrity. This rapid development capability is particularly valuable in agile environments where speed and flexibility are critical.
4. Data Analysis and Reporting
Prisma’s powerful querying capabilities make it an excellent tool for data analysis and reporting within your web application. You can easily retrieve and aggregate data, perform complex calculations, and generate reports based on your application’s data.
For example, if you’re building an e-commerce application, you can use Prisma to generate sales reports, track customer behavior, and analyze inventory levels. The type-safe queries ensure that your data analysis logic is accurate and reliable, helping you make informed business decisions.
5. Microservices Architecture
In modern web development, microservices architecture has become increasingly popular due to its scalability and modularity. Prisma is well-suited for use in microservices, as it allows each service to have its own database schema and Prisma Client, enabling clear separation of concerns.
With Prisma, you can easily manage database interactions within each microservice, ensuring that each service is optimized for its specific needs. This modular approach also makes it easier to scale and maintain your application over time.
Getting Started with Prisma
If you’re interested in using Prisma in your web development projects, getting started is straightforward. Here’s a quick overview of the steps involved:
- Install Prisma: You can install Prisma using npm or yarn:
npm install @prisma/client
npx prisma init
- Set Up Your Database: Configure your database connection in the
prisma/schema.prisma
file. Prisma supports various databases, so you can choose the one that best suits your needs. - Define Your Data Model: In the
schema.prisma
file, define your database schema using Prisma’s intuitive data modeling language. - Generate Prisma Client: Run the following command to generate Prisma Client based on your data model:
npx prisma generate
- Start Writing Queries: You can now use Prisma Client to interact with your database in a type-safe manner.
const users = await prisma.user.findMany();
- Manage Migrations: Use Prisma Migrate to manage and apply schema changes as your application evolves:
npx prisma migrate dev --name init
Conclusion
Prisma is a powerful and versatile tool that can greatly enhance your web development workflow. By providing type-safe database queries, easy migration management, and a user-friendly API, Prisma allows developers to focus on building features rather than wrestling with database intricacies. Whether you’re building APIs, server-side applications, or microservices, Prisma can help you create robust, scalable, and maintainable applications with ease.
As web development continues to evolve, tools like Prisma will play an increasingly important role in helping developers manage the growing complexity of modern applications. If you haven’t already, consider giving Prisma a try in your next project—you might find it to be the game-changer you’ve been looking for.
Related Article
10 Essential Skills Every Web Developer Should Learn
Photo by Joshua Aragon on Unsplash In today’s tech-driven world,... Photo by Joshua Aragon on Unsplash In today’s tech-driven world, web development is one of the most in-demand careers. Whether...
Top Web Development Trends in 2024: What’s New in Tech?
Photo by Andrew Neel on Unsplash The web development landscape... Photo by Andrew Neel on Unsplash The web development landscape is constantly evolving, and 2024 is shaping up to be...
What is SASS in Web Development?
As the web development landscape continues to evolve, developers are... As the web development landscape continues to evolve, developers are constantly seeking tools and methodologies to streamline their workflows, enhance...