What is SASS in Web Development?
ghifari
August 25, 2024
8 min read
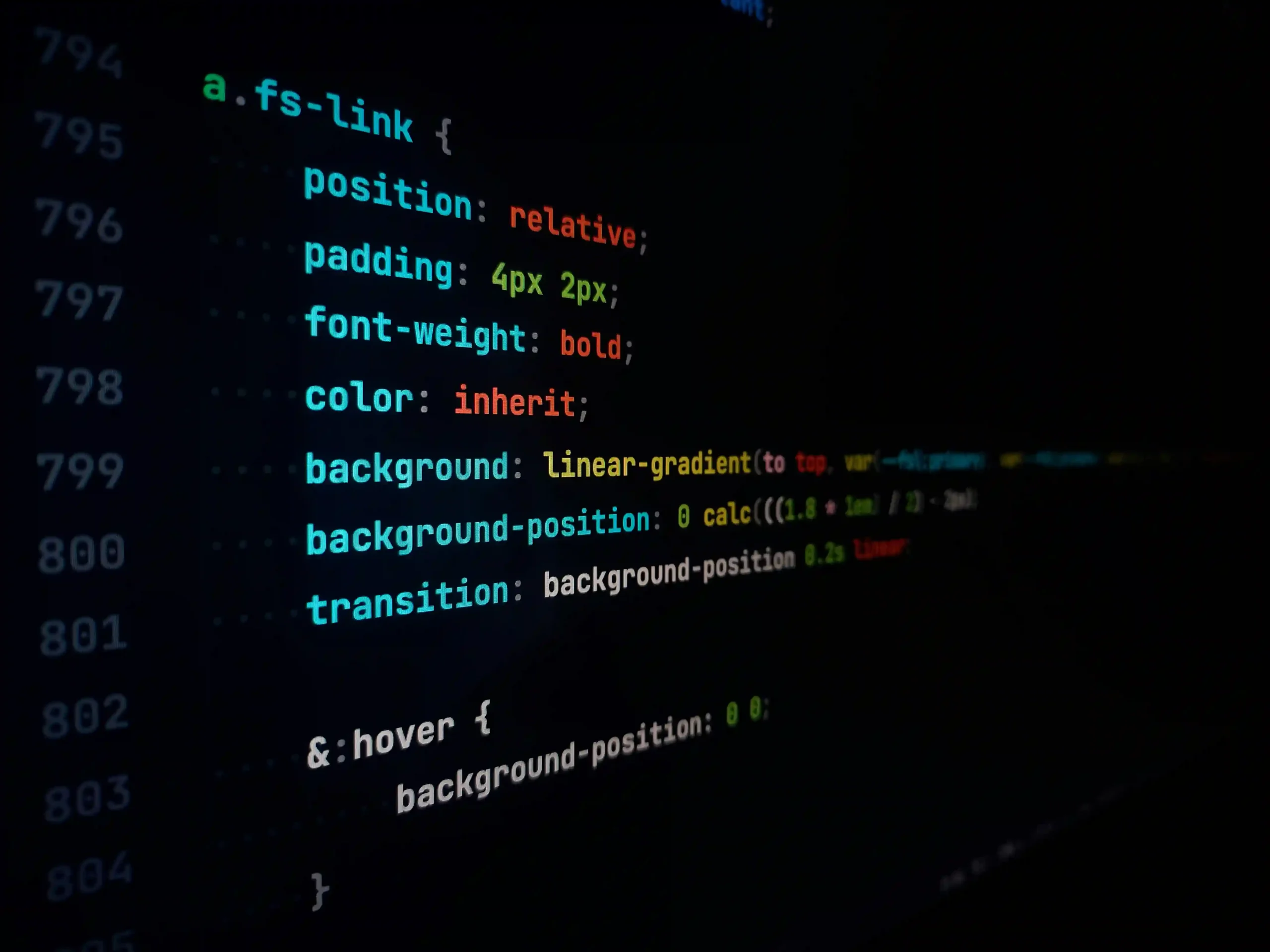
As the web development landscape continues to evolve, developers are constantly seeking tools and methodologies to streamline their workflows, enhance productivity, and deliver better user experiences. One such tool that has gained immense popularity in recent years is SASS (Syntactically Awesome Style Sheets). If you’re new to web development or looking to refine your skills, understanding what SASS is and how it can improve your CSS (Cascading Style Sheets) workflow is crucial.
In this comprehensive guide, we’ll dive deep into the world of SASS, exploring its features, benefits, and how it can revolutionize the way you write CSS. By the end of this article, you’ll have a solid grasp of what SASS is, why it’s so valuable, and how you can start using it in your web development projects.
What is SASS?
SASS, which stands for Syntactically Awesome Style Sheets, is a CSS preprocessor that extends the capabilities of standard CSS. Essentially, SASS allows you to write CSS in a more structured, modular, and efficient way. It introduces features like variables, nested rules, mixins, and functions that are not available in vanilla CSS, making it a powerful tool for developers who want to write cleaner and more maintainable code.
SASS was created by Hampton Catlin and developed by Nathan Weizenbaum in 2006. Since then, it has become one of the most widely used CSS preprocessors, supported by a large and active community. It has two syntax styles: the older, indented syntax (often referred to as SASS) and the newer, more CSS-like syntax known as SCSS (Sassy CSS). Both syntaxes are fully compatible with one another, and you can choose the one that best fits your coding style.
The Difference Between CSS and SASS
To fully appreciate the power of SASS, it’s important to understand the limitations of standard CSS. While CSS is a fundamental part of web development, it has some drawbacks that can make it challenging to work with on larger projects:
- Lack of Variables: CSS does not support variables, meaning you have to repeat the same values throughout your stylesheet. This can lead to redundancy and make it difficult to update values across your entire project.
- No Nesting: CSS requires you to write out selectors in full, even when they are logically nested within each other. This can result in lengthy and hard-to-read code.
- Limited Reusability: CSS lacks built-in mechanisms for reusing code snippets, leading to duplication and inconsistencies.
- No Functions or Logic: CSS is purely declarative and doesn’t allow for any programming logic, making it less flexible for complex styling needs.
SASS addresses these limitations by adding a layer of abstraction and additional functionality on top of CSS. It compiles into standard CSS, which means that any browser that supports CSS can use styles written in SASS.
Read also: Top 10 Tools Every Web Developer Should Know
Key Features of SASS
SASS introduces several powerful features that significantly enhance the CSS development process:
1. Variables
One of the most useful features of SASS is the ability to use variables. Variables allow you to store values (like colors, fonts, or sizes) in one place and reuse them throughout your stylesheet. This makes it easier to maintain and update your styles.
$primary-color: #3498db;
$font-stack: Helvetica, sans-serif;
body {
font-family: $font-stack;
color: $primary-color;
}
In the example above, $primary-color
and $font-stack
are variables that store commonly used values. If you need to change the primary color or font, you can do so by updating the variable, and the change will be applied wherever the variable is used.
2. Nesting
SASS allows you to nest your CSS selectors in a way that follows the same visual hierarchy of your HTML, making your code more readable and organized.
nav {
ul {
margin: 0;
padding: 0;
list-style: none;
li {
display: inline-block;
a {
text-decoration: none;
color: $primary-color;
}
}
}
}
In this example, the nested structure of the SASS code mirrors the structure of the HTML, making it easier to see the relationships between elements.
3. Partials and Import
SASS allows you to split your CSS into smaller, reusable pieces using partials. Partials are SASS files that contain snippets of CSS and are prefixed with an underscore (_
). These partials can then be imported into other SASS files, keeping your code modular and maintainable.
// _variables.scss
$primary-color: #3498db;
$secondary-color: #2ecc71;
// _base.scss
body {
font-family: Arial, sans-serif;
color: $primary-color;
}
// main.scss
@import 'variables';
@import 'base';
In the example above, _variables.scss
and _base.scss
are partials that are imported into main.scss
. This approach allows you to organize your styles into logical sections, making your codebase easier to manage.
4. Mixins
Mixins are another powerful feature of SASS that allow you to create reusable blocks of code. Mixins can take arguments, making them highly flexible and customizable.
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
-ms-border-radius: $radius;
border-radius: $radius;
}
.box {
@include border-radius(10px);
}
The border-radius
mixin in the example above can be reused across your stylesheet, ensuring consistency and reducing the amount of code you have to write.
5. Inheritance (Extend/Inheritance)
SASS provides a feature called @extend
that allows one selector to inherit the styles of another. This is particularly useful when you want to avoid duplicating code.
%message {
padding: 10px;
border: 1px solid #ccc;
}
.success {
@extend %message;
border-color: green;
}
.error {
@extend %message;
border-color: red;
}
Here, %message
is a placeholder selector that defines some common styles. The .success
and .error
classes extend %message
, inheriting its styles and adding their own.
6. Functions
SASS allows you to define your own functions to perform calculations and manipulate values. This can be particularly useful for generating dynamic styles.
@function calculate-spacing($base-spacing, $multiplier) {
@return $base-spacing * $multiplier;
}
.container {
padding: calculate-spacing(10px, 2);
}
In this example, the calculate-spacing
function takes two arguments and returns the product, which is then used to set the padding of the .container
element.
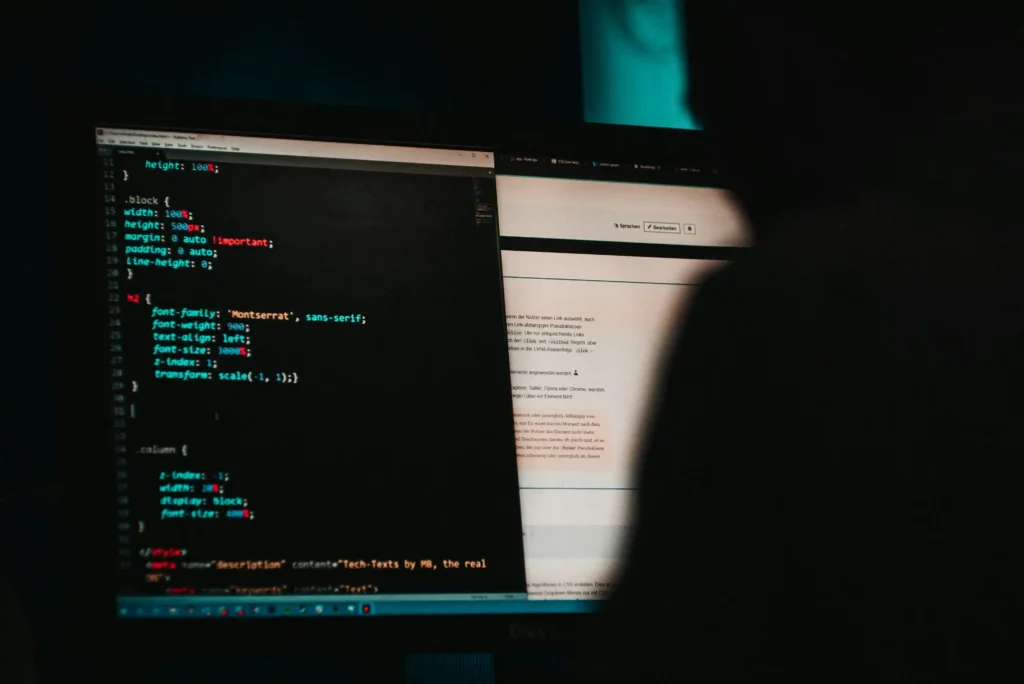
Why Use SASS?
Now that we’ve covered what SASS is and some of its key features, let’s explore why you might choose to use SASS in your web development projects.
1. Improved Code Organization
SASS encourages better code organization through its support for variables, nesting, partials, and mixins. This modular approach to styling makes your codebase more manageable, especially in larger projects.
2. DRY Principle (Don’t Repeat Yourself)
SASS helps you adhere to the DRY principle by allowing you to reuse code through variables, mixins, and inheritance. This reduces redundancy and makes your stylesheets more efficient and easier to maintain.
3. Easier Maintenance and Scalability
With SASS, updating your styles is easier and less error-prone. Changes can be made in one place (e.g., a variable or mixin) and applied consistently across your entire project. This makes your code more scalable as your project grows.
4. Enhanced Productivity
SASS’s powerful features, such as functions and mixins, automate repetitive tasks and reduce the amount of code you need to write. This speeds up your development process and allows you to focus on more important aspects of your project.
5. Compatibility with CSS
SASS compiles into standard CSS, which means it works in all browsers that support CSS. You don’t have to worry about compatibility issues, and you can start using SASS in your existing projects without any major changes.
How to Get Started with SASS
If you’re ready to start using SASS in your web development projects, here’s a quick guide to getting started:
1. Install SASS
To use SASS, you’ll need to install it on your development environment. SASS is written in Ruby, so you can install it via RubyGems. If you’re using Node.js, you can also install it via npm.
# Install SASS via RubyGems
gem install sass
# Install SASS via npm
npm install -g sass
2. Create a SASS File
Once SASS is installed, you can create a new SASS file with the .scss
or .sass
extension. Write your styles using the SASS syntax, taking advantage of features like variables, nesting, and mixins.
// style.scss
$primary-color: #3498db;
body {
color: $primary-color;
font-family: Arial, sans-serif;
}
3. Compile SASS to CSS
To use your SASS styles in a web project, you’ll need to compile them into standard CSS. SASS provides a command-line tool that automatically compiles your SASS files into CSS.
# Compile a single SASS file
sass styles.scss styles.css
# Watch a directory for changes and automatically compile SASS to CSS
sass --watch scss:css
4. Integrate SASS into Your Workflow
SASS can be integrated into your existing development workflow in various ways. If you’re using a task runner like Gulp or Grunt, or a build tool like Webpack, you can configure it to automatically compile your SASS files whenever they change.
5. Explore Advanced Features
As you become more comfortable with SASS, explore its more advanced features, such as custom functions, loops, and conditionals. These tools can help you write even more dynamic and flexible styles.
Conclusion
SASS is an invaluable tool for modern web development, offering a wide range of features that improve code organization, maintainability, and productivity. Whether you’re working on a small personal project or a large enterprise application, SASS can help you write cleaner, more efficient CSS that’s easier to maintain and scale.
By adopting SASS, you’ll not only streamline your workflow but also enhance the quality of your code, leading to better user experiences and more successful projects.
If you’re looking to elevate your web development projects with professional expertise, Available Dev is here to help. We offer comprehensive web development services tailored to meet your needs. Whether you’re building a new website from scratch or optimizing an existing one, our team of experienced developers is ready to assist you every step of the way. Contact us today and let’s create something awesome together!
Related Article
10 Essential Skills Every Web Developer Should Learn
Photo by Joshua Aragon on Unsplash In today’s tech-driven world,... Photo by Joshua Aragon on Unsplash In today’s tech-driven world, web development is one of the most in-demand careers. Whether...
Top Web Development Trends in 2024: What’s New in Tech?
Photo by Andrew Neel on Unsplash The web development landscape... Photo by Andrew Neel on Unsplash The web development landscape is constantly evolving, and 2024 is shaping up to be...
How to Outsource Web Development
In today’s fast-paced digital world, having a strong online presence... In today’s fast-paced digital world, having a strong online presence is essential for any business. A well-designed website can serve...